Python Text Strings and Regular Expressions Syllabus
Intensive Short Course on Python Text Strings and Regular Expressions
Data Science is far more than just visualizing data and analyzing it by means of sophisticated statistical algorithms. Data first need to be collected (usually extracted from large text files), cleaned, and organized. Python provides powerful operations with text strings and regular expressions to do that.
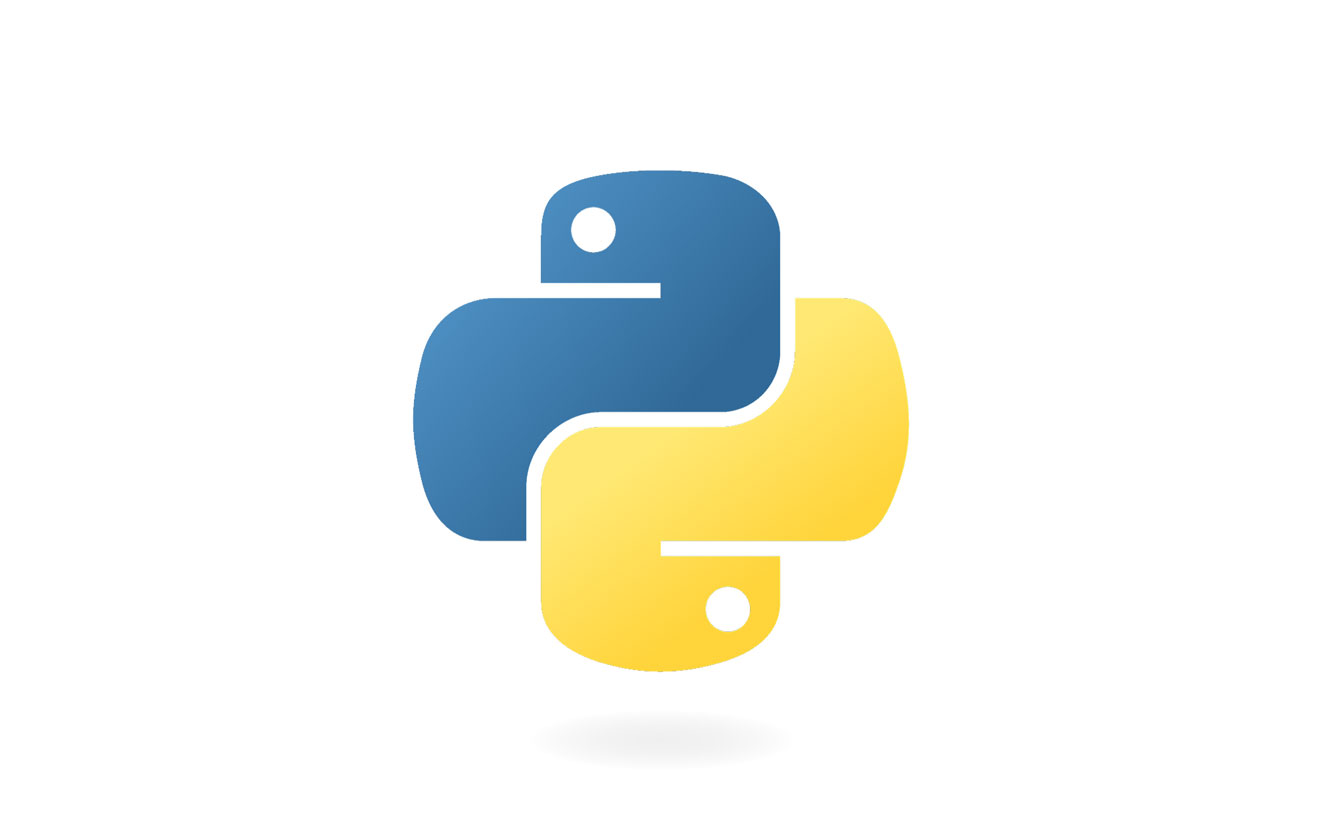
What You Will Learn
This intensive short course will teach you how to work with text strings and regular expressions in Python.
Recommended Background
To make the most of this course, you should have a basic understanding of Python structures and procedures. You will be using functions, conditions, lists and the for loop. We will review these as we go, but the presentation will not be as detailed as the Intro to Python courses – simply because our focus is on text strings.
If you are a complete novice to Python, you are welcome to give it a try anyway. Should you find this course too difficult, we recommend taking Python Fundamentals first.
Course Structure and Length
This course is self-paced, and you will practice each skill and concept as you go. Automatic feedback is built into the course for both practices and quizzes. The course is divided into five Sections. Each Section consists of 7 instructional/practice levels, a quiz, and a master (proficiency) level. You can return to any level or quiz for review.
Python Text Strings and Regular Expressions is designed to take approximately 20 hours. Since the course is self-paced, the amount of time required to complete the course will vary from student to student. You are responsible for learning both the tutorial content and the skills acquired through practice.
Section 1
- Use single and double quotes to enclose text strings.
- Identify trailing spaces using the built-in function repr(). Clean text of trailing spaces.
- Compare text strings with the == operator, whose result is either True or False.
- Print strings using the built-in function print() with optional parameters sep and end.
- Obtain help with a keyword or function using the built-in function help() .
- Write docstrings for your own functions, which will be detected by help().
- Write functions for strings as part of a program.
- Add and multiply strings using positive integers.
- Update strings with the operators += and *=.
- Understand that Python is an interpreted, dynamically (weakly) typed language. It is case-sensitive.
- Learn elements of style as outlined in the PEP8 — Style Guide for Python Code.
Section 2
- Combine single and double quotes in one text string.
- Obtain the length of text strings with the function len().
- Work with the special characters \n, \” and \’.
- Know that these special characters are text strings of length 1, same as regular text characters.
- Cast numbers to text strings with the function str(), and insert numbers into text strings.
- Cast text strings to numbers with the functions int() and float().
- Display the type of variables, and check the type of variables at runtime.
- Use the text string methods lower(), upper() and title().
- Know that methods do not change the original text string.
- Clean text strings, automatically eliminate trailing spaces and unwanted special characters, using the methods rstrip(), lstrip() and strip().
- Split a text string into a list of words with the method split().
- Check for substrings using the keyword in.
- Make a text search case-insensitive by first lowercasing both text strings.
- Count the occurrences of substrings in text strings with the method count().
- Understand the difference between a function and a method.
- Use conditions and for loops with text strings.
Section 3
- Search for and replace substrings in text strings with the method replace().
- Count the occurrences of substrings in text strings with the method count().
- Zip two lists and use the for loop to parse them at the same time.
- Erase parts of text strings by replacing them with the empty string ”.
- Clean text strings of unwanted characters.
- Shrink large empty spaces in text strings.
- Swap the contents of two text strings.
- Swap two substrings in a text string.
- Learn about the ROT13 cipher and write your own Morse code translator.
- Use Python functions returning multiple values, the while loop and the break statement.
Section 4
- Understand that text strings are immutable objects in Python.
- Access individual characters in text strings via their indices.
- Slice text strings and reverse them using slicing.
- Use system date and time in your programs.
- Obtain the position of a substring in a given text string.
- Understand how text strings are represented in computer memory.
- Access ASCII/Unicode codes of text characters.
- Access ASCII/Unicode characters via their codes.
- Compare text strings using the operators < , <= , > , >= like numbers.
- Create text strings with characters which are not present on your keyboard.
- Implement a text search based on a simple regular expression.
Section 5
- Understand regular expressions and where they are used.
- Become familiar with available functionality in Python’s regular expressions module re.
- Use functions search(), match() and findall().
- Work with greedy and non-greedy repeating patterns.
- Use character classes and groups of characters.
- Work with the most important metacharacters and special sequences.
- Solve two tasks of practical importance: extract file names and email addresses from given text data.